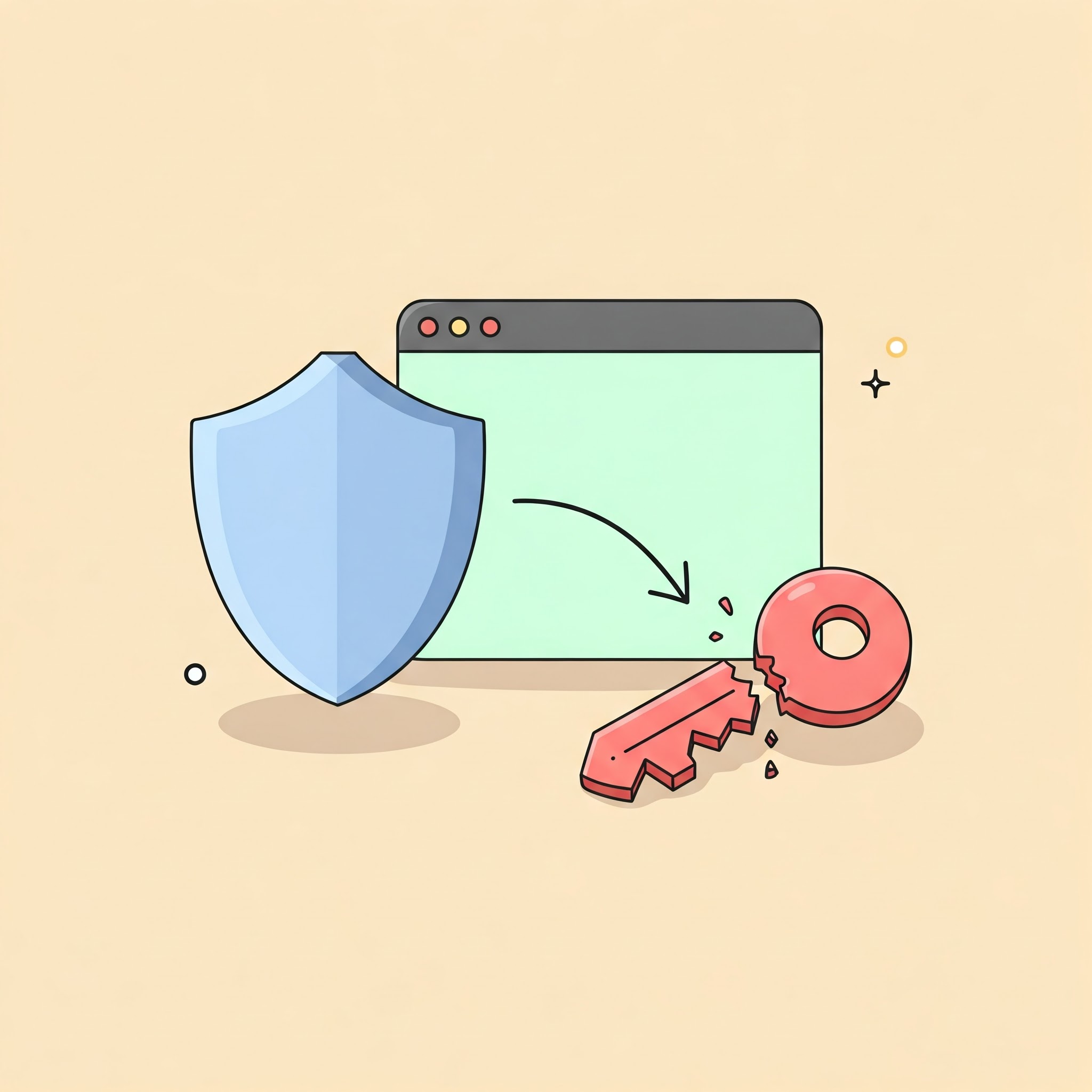
Building dynamic web applications with frameworks like React, Vue, Angular, or Svelte frequently involves integrating third-party services through APIs. These integrations often hinge on API keys for authentication. When you first get that key, a critical question arises: where does it safely reside in your project?
A seemingly straightforward path might tempt you: embedding the key directly within your frontend JavaScript code – maybe in component state, a config object, or an environment file. This approach, however common for beginners, opens a gaping security hole. It's a shortcut that bypasses essential security practices.
This article unpacks why exposing secret API keys on the client-side is a flawed strategy and details the robust, server-centric approach you should adopt instead.
The Fundamental Flaw: The Browser is Public Ground
The non-negotiable truth is that client-side JavaScript executes in an environment you don't control: the user's browser. Everything required to render and operate your frontend – HTML, CSS, and all the JavaScript logic – is transmitted to and processed by the browser.
This inherently means your frontend code is transparent. Anyone can use browser developer tools to inspect the source files, monitor network traffic, and analyze application data. Any "secret" embedded within this client-side package ceases to be secret the moment it's downloaded. Placing a sensitive API key here is akin to broadcasting your private credentials over a public network – fundamentally unsafe.
The Cascade of Risks from Exposed Keys
Treating an API key casually in your frontend code isn't just poor practice; it invites tangible negative consequences, irrespective of your chosen framework:
- Identity Hijacking: Malicious actors can grab the key and make API calls impersonating your application, potentially performing unauthorized actions.
- Uncontrolled Billing Explosions: If the API is metered or paid, you foot the bill for all usage tied to that key. An exposed key can lead to staggering, unforeseen costs generated by others.
- Service Denial via Quota Depletion: Attackers can intentionally exhaust your API usage quotas (whether free or paid), effectively blocking your legitimate users from accessing essential features.
- Compromised Data Security: Depending on the permissions granted by the key, its exposure could lead to unauthorized access or manipulation of sensitive data.
- Breaching Provider Trust: Most API providers explicitly prohibit embedding secret keys client-side in their terms of service. Violating this can result in your key being invalidated or your entire account suspended.
The Secure Standard: Your Server as the Key Guardian
So, how do you use API keys without dangerously exposing them? The professional standard involves delegating the responsibility to your backend. Your server acts as a secure intermediary or guardian for the secret key.
Here's the secure workflow:
- Frontend Requests Your Backend: Your React/Vue/Angular component initiates a request, not to the external API directly, but to a specific endpoint you've created on your own backend server (e.g.,
/api/proxy/service-name
). - Backend Securely Accesses the Key: Your backend code (Node.js, Python, Ruby, Java, PHP, Go, etc., or a serverless function) receives this request. It then securely retrieves the actual secret API key from its protected storage – typically server-side environment variables or a dedicated secrets management system. Crucially, the secret key never transits to the browser.
- Backend Communicates with External API: Armed with the secret key, your backend server makes the necessary call to the third-party API service.
- Backend Relays Result to Frontend: The external API responds to your backend. Your backend then processes (if necessary) and forwards the relevant result back to your frontend application.
The Secure Path
This isolates the secret, adding a vital layer of security and control.
Demystifying .env
Files and Build-Time Injection (REACT_APP_
, VITE_
, etc.)
Environment variables in frontend projects often cause confusion. Tools like Create React App (REACT_APP_
), Vite (VITE_
), and Next.js (NEXT_PUBLIC_
) use specific prefixes to identify variables that should be injected directly into the client-side JavaScript bundle during the build process.
Understand this clearly: these prefixed variables are designed for public configuration, not secret storage. They make values accessible in the browser code, meaning they become public knowledge.
- Use them for: Your backend's URL, public tracking IDs, non-sensitive feature flags.
- Never use them for: Secret API keys, passwords, private tokens, or any credential that must remain confidential. Relying on them for secrets provides zero actual security.
Conclusion: Build Security In, Not As An Afterthought
Handling API keys securely is not an optional extra; it's a fundamental aspect of professional web development. The allure of quick integration must never overshadow the need to protect sensitive credentials.
By consistently implementing the server-side guardian pattern – keeping secret keys strictly within your controlled backend environment – you build more robust, trustworthy, and resilient applications. Make this secure approach your default. Your users, your clients, and your future self (avoiding security incidents!) will thank you.
Disclaimer: This article provides information on common security practices. While aiming for originality in expression, the underlying technical concepts are widely established in the software development field. Always consult the specific documentation and terms of service for the APIs you use.
Posted By:

UCodeSoft